Truffle
In this tutorial, we'll walk through creating a basic Truffle project and deploying a token contract.
Here's a video walkthrough:
Prerequisitesβ
Before you begin, ensure you've:
- Set up your wallet
- Funded your wallet with Linea ETH on either the testnet or mainnet
- Installed Truffle using the recommended installation procedure.
Create a Truffle projectβ
To create an empty Truffle project, run:
truffle init linea-tutorial
Change into the new directory:
cd linea-tutorial
Write the smart contractβ
Create your smart contract in the contracts
directory by either creating a new file Token.sol
or calling truffle create contract Token
. Then, add the following code:
pragma solidity 0.8.17;
// SPDX-License-Identifier: MIT
contract Token {
string public name = "My Token";
string public symbol = "MTK";
uint8 public decimals = 18;
uint256 public totalSupply = 100000000;
mapping (address => uint256) public balances;
address public owner;
constructor() {
owner = msg.sender;
balances[owner] = totalSupply;
}
function transfer(address recipient, uint256 amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance.");
balances[msg.sender] -= amount;
balances[recipient] += amount;
}
}
You can check if it compiles by running truffle compile
from the root folder.
Write the migration scriptβ
To tell Truffle how, and in what order we want to deploy our smart contracts, we need to write a migration script.
Create 1_deploy_token.js
in the migrations
directory, and add the following code:
const Token = artifacts.require("Token");
module.exports = function (deployer) {
deployer.deploy(Token);
};
Deploy your contractβ
Truffle allows you to deploy through the Truffle Dashboard using your MetaMask wallet!
Truffle Dashboardβ
Truffle Dashboard allows you to forgo saving your private keys locally, instead connecting to your MetaMask wallet for deployments. To deploy with Truffle Dashboard, you need to:
- Run
truffle dashboard
in your terminal, which will open a window on port24012
. - Navigate to
localhost:24012
in your browser. Please ensure that Dashboard is connected to the Linea testnet by connecting your MetaMask wallet to Linea. For reference, the Linea testnet network ID is59140
.
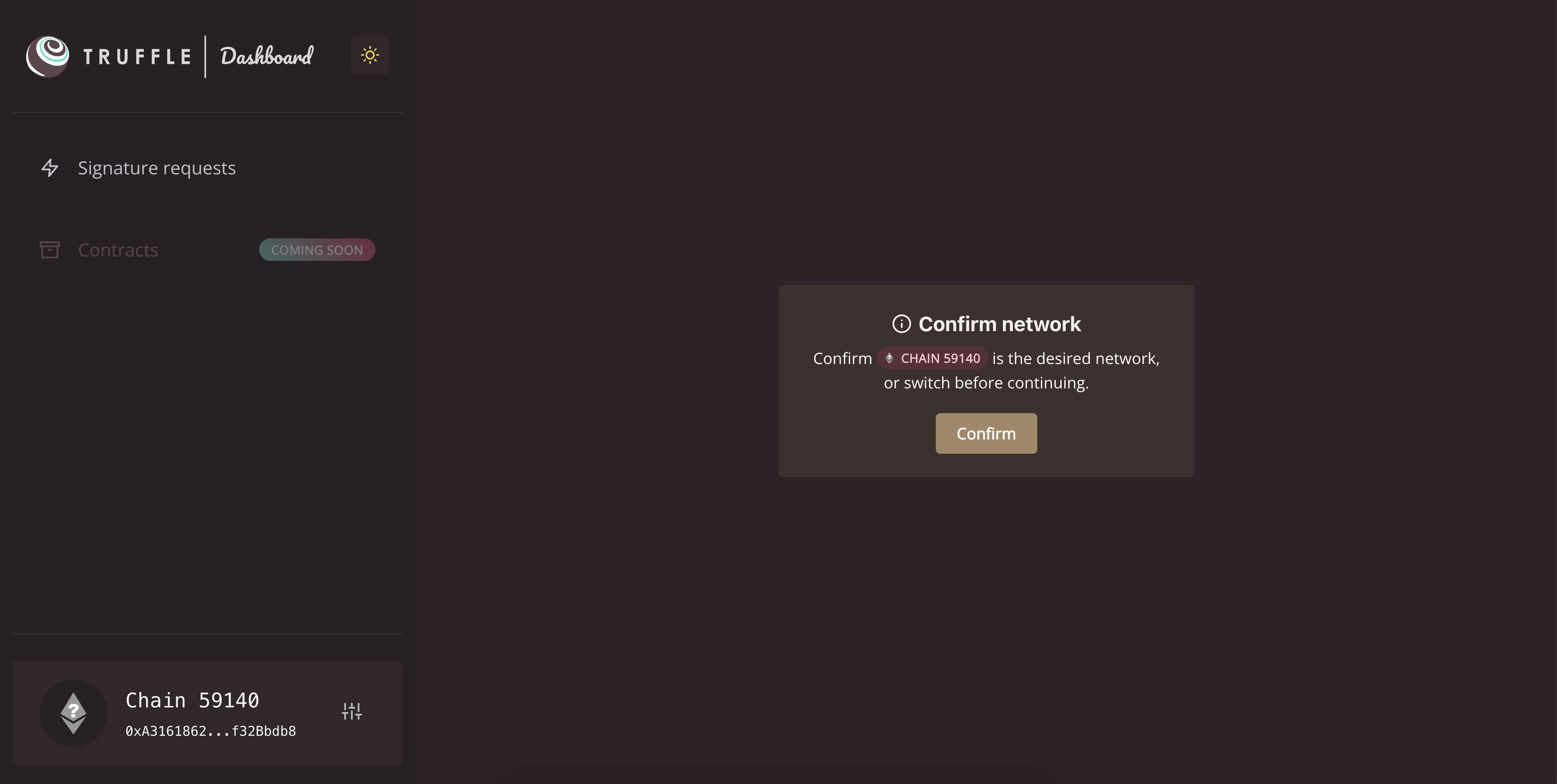
- Run
truffle migrate --network dashboard
in a separate terminal. - Navigate back to
localhost:24012
. You should see a prompt asking you to confirm the deployment. Click Confirm.
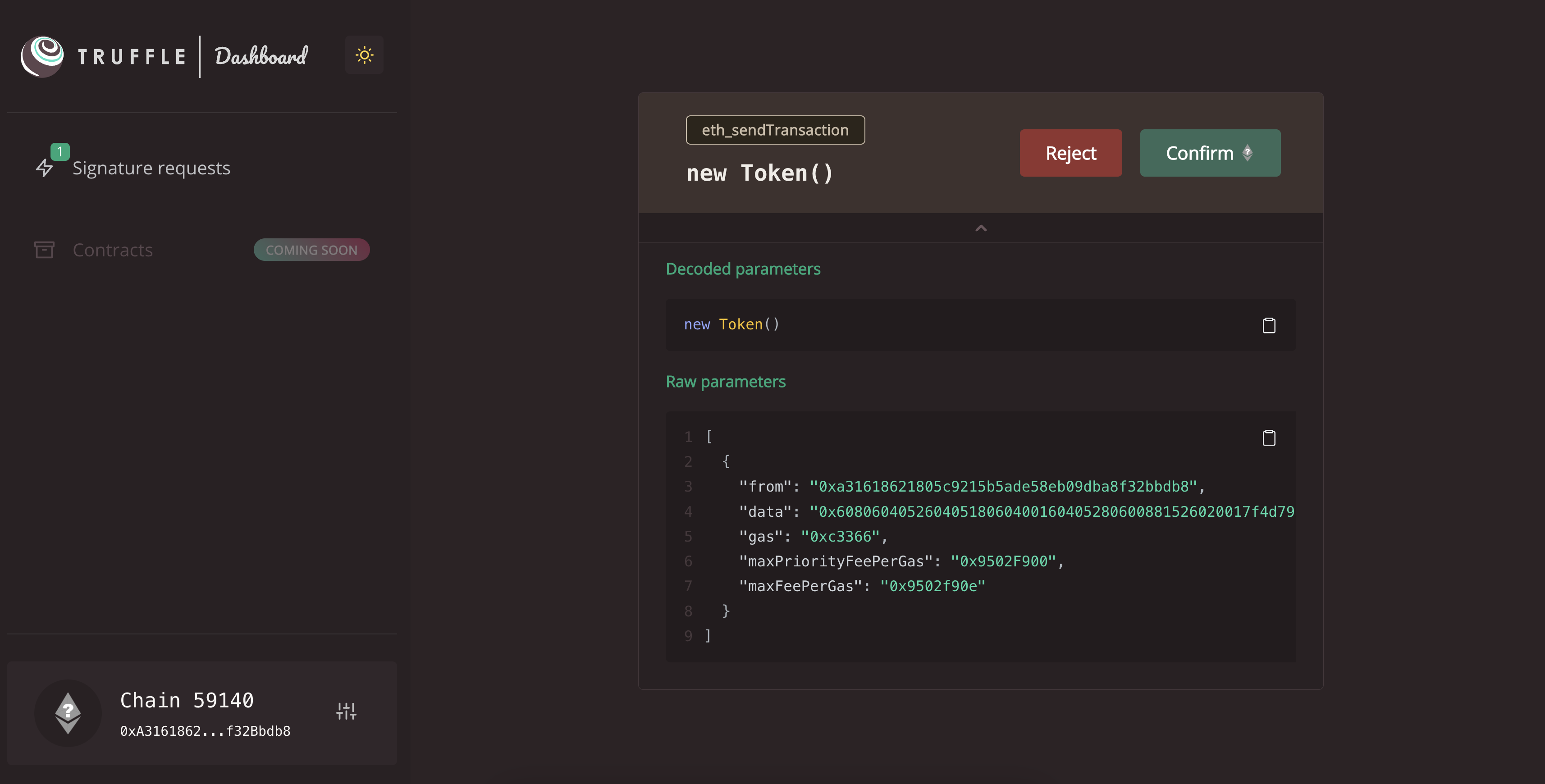
truffle-config.jsβ
You can deploy with Truffle using the command line, by specifying the Linea in truffle-config.js
. To do so, you need to:
Create a
.env
file in the root folder with your wallet's mnemonic. This is your secret recovery phrase.MNEMONIC=<MNEMONIC>
dangerPlease do not check your keys into source control. Please add
.env
to your.gitignore
Download
dotenv
and@truffle/hdwallet-provider
npm i -D dotenv
npm i -D @truffle/hdwallet-providerAdd the Linea testnet to your
truffle-config.js
file:- Infura
- Public Endpoint
To use Infura, you'll need to get an API key. Add it to the
.env
file as follows:MNEMONIC=MNEMONIC
INFURA_API_KEY=INFURA_API_KEYThen, modify
truffle-config.js
like so:require("dotenv").config();
const { MNEMONIC, INFURA_API_KEY } = process.env;
const HDWalletProvider = require("@truffle/hdwallet-provider");
module.exports = {
networks: {
linea_testnet: {
provider: () => {
return new HDWalletProvider(
MNEMONIC,
`https://linea-goerli.infura.io/v3/${INFURA_API_KEY}`,
);
},
network_id: "59140",
},
linea_mainnet: {
provider: () => {
return new HDWalletProvider(
MNEMONIC,
`https://linea-mainnet.infura.io/v3/${INFURA_API_KEY}`,
);
},
network_id: "59140",
},
},
// ... rest of truffle-config.js
};The public endpoints are rate limited and not meant for production systems. However, you can use the public endpoints by modifying
hardhat.config.js
as follows:require("dotenv").config();
const { MNEMONIC } = process.env;
const HDWalletProvider = require("@truffle/hdwallet-provider");
module.exports = {
networks: {
linea_testnet: {
provider: () => {
return new HDWalletProvider(
MNEMONIC,
`https://rpc.goerli.linea.build/`,
);
},
network_id: "59140",
},
linea_mainnet: {
provider: () => {
return new HDWalletProvider(
MNEMONIC,
`https://rpc.linea.build/`,
);
},
network_id: "59140",
},
},
// ... rest of truffle-config.js
};Call
truffle migrate --network linea_testnet
ortruffle migrate --network linea_mainnet
from the CLI.Your output should look similar to the following:
Compiling your contracts...
===========================
> Everything is up to date, there is nothing to compile.
Starting migrations...
======================
> Network name: 'linea'
> Network id: 59140
> Block gas limit: 30000000 (0x1c9c380)
1_deploy_token.js
=================
Deploying 'Token'
-----------------
> transaction hash: 0x412d58eaf4cc387fe1efa52b105f6fadac36db934b1617d04eaefc1947197525
> Blocks: 0 Seconds: 0
> contract address: 0x33b4D321Fc300E4f402820052EFA0958272D2AE5
> block number: 143419
> block timestamp: 1677366505
> account: YOUR_ACCOUNT_NUMBER
> balance: 0.088400819995522296
> gas used: 639672 (0x9c2b8)
> gas price: 2.500000007 gwei
> value sent: 0 ETH
> total cost: 0.001599180004477704 ETH
> Saving artifacts
-------------------------------------
> Total cost: 0.001599180004477704 ETH
Summary
=======
> Total deployments: 1
> Final cost: 0.001599180004477704 ETH
``` -->
Next, you can optionally verify your contract on the network.